Well, as web developers, we are always dealing with JSON data which are coming from different sources. Either we are serializing our own entities or it's coming from the external sources like third-party services and so on.
If the data is coming from an external source, one common requirement is to always de-serialize it back to the data model to be able to process the data. Creating data model for JSON data is not the most exciting work in the world. Specially when the data model is a bit nested and complex.
Fortunately there is a very nice feature is Visual Studio which makes the life much easier. This feature is called Paste Special.
To take advantage of this feature you need to first Copy the JSON data . Imaging there is JSON data as follow:
{ "glossary": { "title": "example glossary", "GlossDiv": { "title": "S", "GlossList": { "GlossEntry": { "ID": "SGML", "SortAs": "SGML", "GlossTerm": "Standard Generalized Markup Language", "Acronym": "SGML", "Abbrev": "ISO 8879:1986", "GlossDef": { "para": "A meta-markup language, used to create markup languages such as DocBook.", "GlossSeeAlso": ["GML", "XML"] }, "GlossSee": "markup" } } } } }
And we require to create the data model for that. To do so, just Copy the data in memory and go to Visual Studio, create a new class (or an existing one where we intend to have our data model).
From the Edit menu in Visual Studio, select the Paste Special and from the sub menu, Paste JSON As Classes.
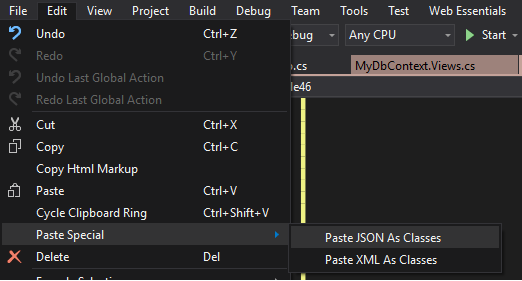
Then, the data model will be generated as follow:
public class Rootobject { public Glossary glossary { get; set; } } public class Glossary { public string title { get; set; } public Glossdiv GlossDiv { get; set; } } public class Glossdiv { public string title { get; set; } public Glosslist GlossList { get; set; } } public class Glosslist { public Glossentry GlossEntry { get; set; } } public class Glossentry { public string ID { get; set; } public string SortAs { get; set; } public string GlossTerm { get; set; } public string Acronym { get; set; } public string Abbrev { get; set; } public Glossdef GlossDef { get; set; } public string GlossSee { get; set; } } public class Glossdef { public string para { get; set; } public string[] GlossSeeAlso { get; set; } }
Here we go! As you see the initial data model has been generated for us and we just need to make our customizations.
As you may have noticed, there is one more option under Paste Special menue item, named Paste XML As Classes. This item does the same thing but for XML data. That means you just need to copy your XML data to the memory and from the Paste Special menu item, choose Paste XML As Classes this time, to have your data model generated.